Setting up CI
Introduction
Playwright tests can be run on any CI provider. In this section we will cover running tests on GitHub using GitHub actions. If you would like to see how to configure other CI providers check out our detailed doc on Continuous Integration.
You will learn
Setting up GitHub Actions
To add a GitHub Actions file first create .github/workflows
folder and inside it add a playwright.yml
file containing the example code below so that your tests will run on each push and pull request for the main/master branch.
name: Playwright Tests
on:
push:
branches: [ main, master ]
pull_request:
branches: [ main, master ]
jobs:
test:
timeout-minutes: 60
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v4
- name: Set up Python
uses: actions/setup-python@v4
with:
python-version: '3.11'
- name: Install dependencies
run: |
python -m pip install --upgrade pip
pip install -r requirements.txt
- name: Ensure browsers are installed
run: python -m playwright install --with-deps
- name: Run your tests
run: pytest --tracing=retain-on-failure
- uses: actions/upload-artifact@v4
if: ${{ !cancelled() }}
with:
name: playwright-traces
path: test-results/
To learn more about this, see "Understanding GitHub Actions".
Looking at the list of steps in jobs.test.steps
, you can see that the workflow performs these steps:
- Clone your repository
- Install language dependencies
- Install project dependencies and build
- Install Playwright Browsers
- Run tests
Create a Repo and Push to GitHub
Once you have your GitHub actions workflow setup then all you need to do is Create a repo on GitHub or push your code to an existing repository. Follow the instructions on GitHub and don't forget to initialize a git repository using the git init
command so you can add, commit and push your code.
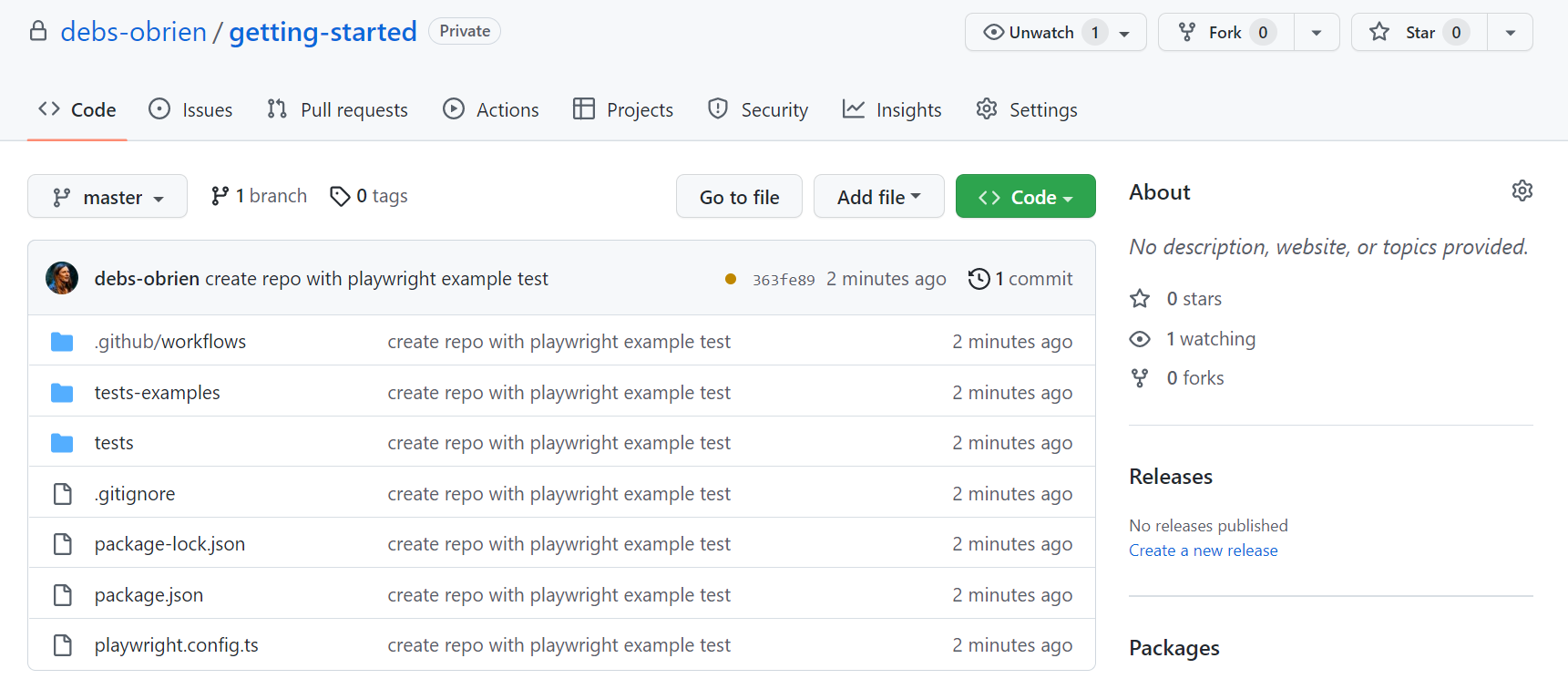
Opening the Workflows
Click on the Actions tab to see the workflows. Here you will see if your tests have passed or failed.
Viewing Test Logs
Clicking on the workflow run will show you the all the actions that GitHub performed and clicking on Run Playwright tests will show the error messages, what was expected and what was received as well as the call log.
Viewing the Trace
trace.playwright.dev is a statically hosted variant of the Trace Viewer. You can upload trace files using drag and drop.