Emulation
Introduction
With Playwright you can test your app on any browser as well as emulate a real device such as a mobile phone or tablet. Simply configure the devices you would like to emulate and Playwright will simulate the browser behavior such as "userAgent"
, "screenSize"
, "viewport"
and if it "hasTouch"
enabled. You can also emulate the "geolocation"
, "locale"
and "timezone"
for all tests or for a specific test as well as set the "permissions"
to show notifications or change the "colorScheme"
.
Devices
Playwright can emulate various devices by specifying setDeviceScaleFactor
, setHasTouch
, setIsMobile
, setScreenSize
, setUserAgent
and setViewportSize
options when creating a context with Browser.newContext().
Viewport
The viewport is included in the device but you can override it for some tests with Page.setViewportSize().
Test file:
The same works inside a test file.
// Create context with given viewport
BrowserContext context = browser.newContext(new Browser.NewContextOptions()
.setViewportSize(1280, 1024));
// Resize viewport for individual page
page.setViewportSize(1600, 1200);
// Emulate high-DPI
BrowserContext context = browser.newContext(new Browser.NewContextOptions()
.setViewportSize(2560, 1440)
.setDeviceScaleFactor(2);
isMobile
Whether the meta viewport tag is taken into account and touch events are enabled.
BrowserContext context = browser.newContext(new Browser.NewContextOptions()
.isMobile(false));
Locale & Timezone
Emulate the user Locale and Timezone which can be set globally for all tests in the config and then overridden for particular tests.
BrowserContext context = browser.newContext(new Browser.NewContextOptions()
.setLocale("de-DE")
.setTimezoneId("Europe/Berlin"));
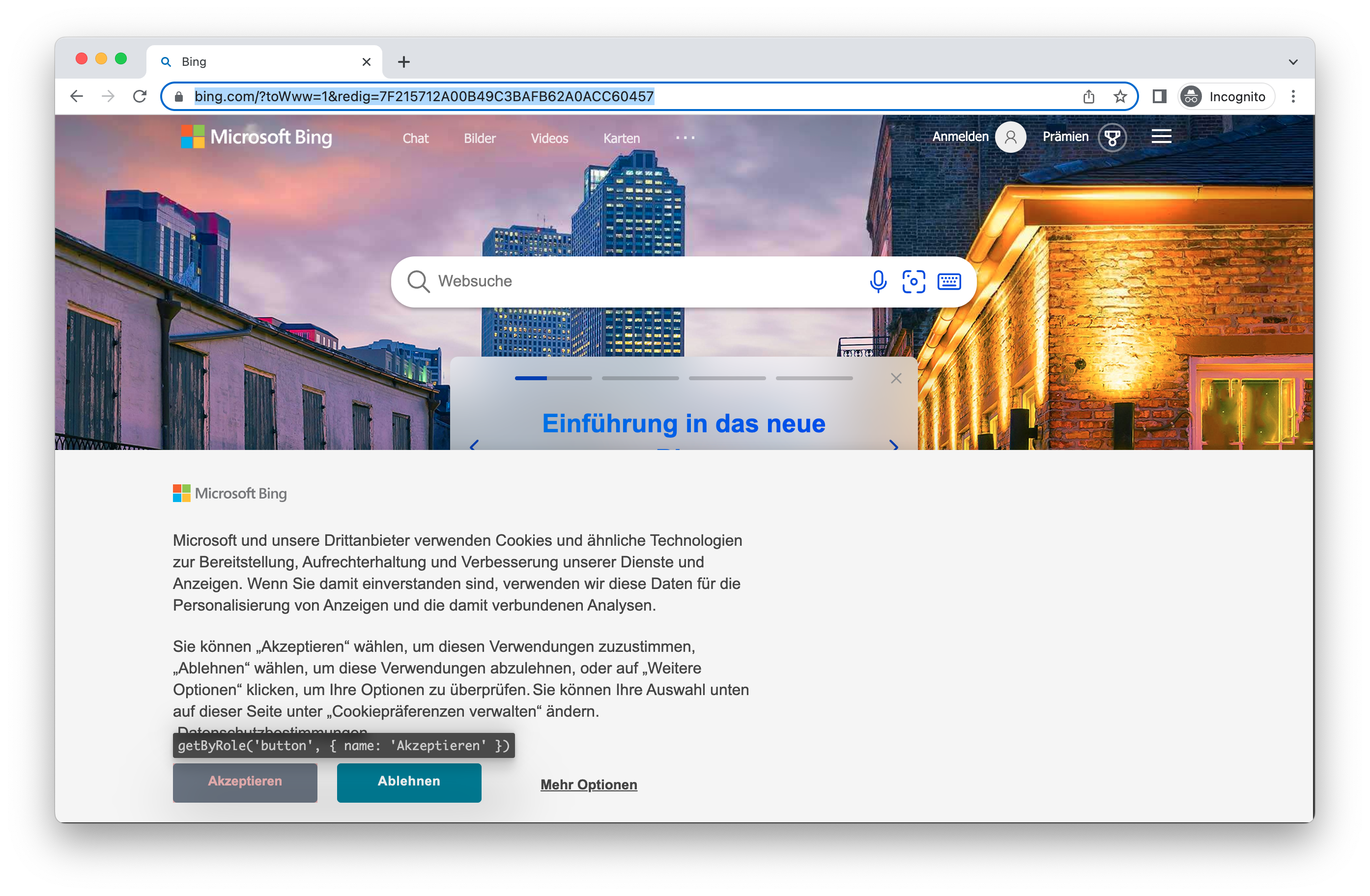
Permissions
Allow app to show system notifications.
BrowserContext context = browser.newContext(new Browser.NewContextOptions()
.setPermissions(Arrays.asList("notifications"));
Allow notifications for a specific domain.
context.grantPermissions(Arrays.asList("notifications"),
new BrowserContext.GrantPermissionsOptions().setOrigin("https://skype.com"));
Revoke all permissions with BrowserContext.clearPermissions().
context.clearPermissions();
Geolocation
Grant "geolocation"
permissions and set geolocation to a specific area.
BrowserContext context = browser.newContext(new Browser.NewContextOptions()
.setGeolocation(41.890221, 12.492348)
.setPermissions(Arrays.asList("geolocation")));
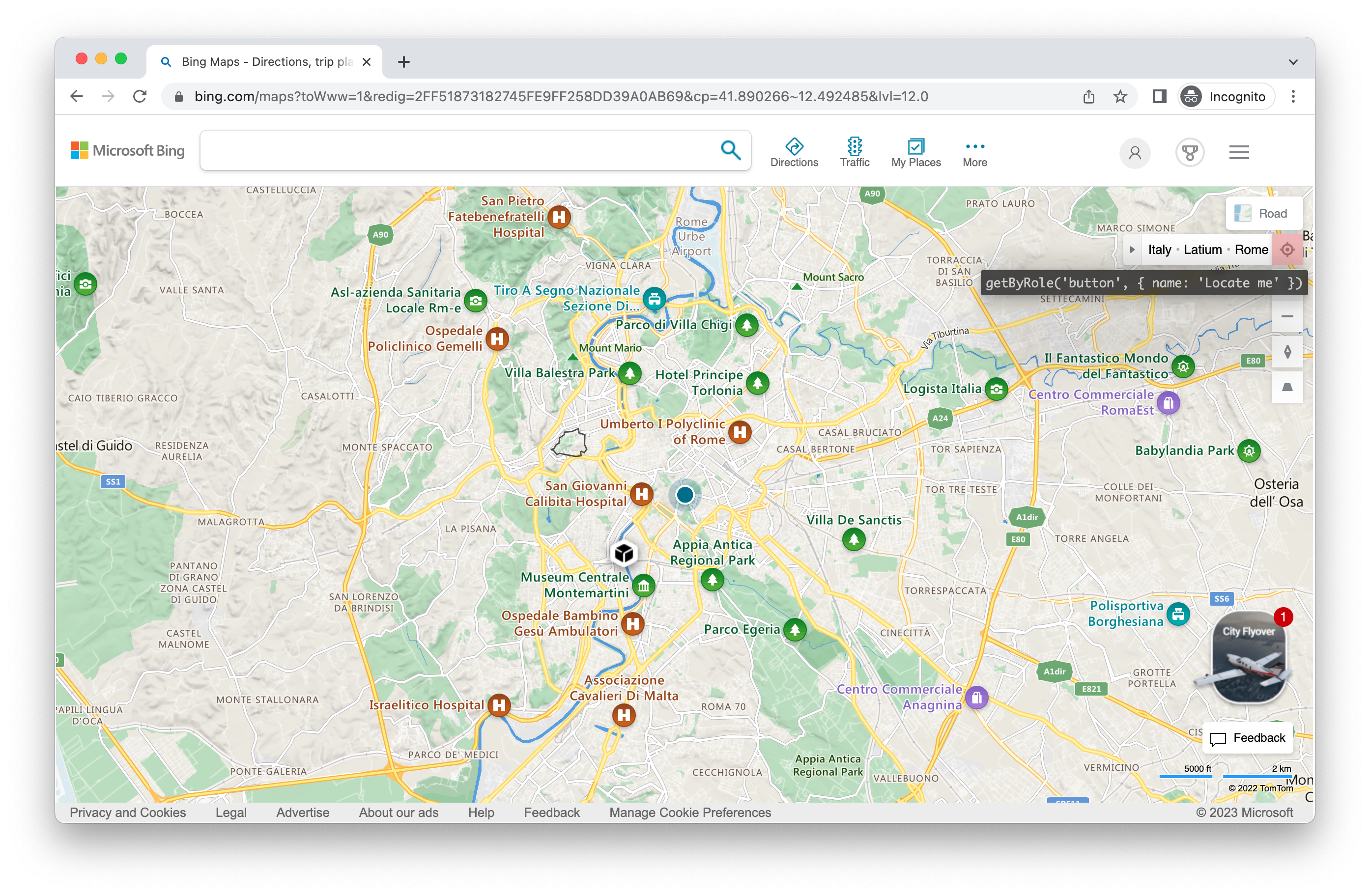
Change the location later:
context.setGeolocation(new Geolocation(48.858455, 2.294474));
Note you can only change geolocation for all pages in the context.
Color Scheme and Media
Emulate the users "colorScheme"
. Supported values are 'light', 'dark', 'no-preference'. You can also emulate the media type with Page.emulateMedia().
// Create context with dark mode
BrowserContext context = browser.newContext(new Browser.NewContextOptions()
.setColorScheme(ColorScheme.DARK)); // or "light"
// Create page with dark mode
Page page = browser.newPage(new Browser.NewPageOptions()
.setColorScheme(ColorScheme.DARK)); // or "light"
// Change color scheme for the page
page.emulateMedia(new Page.EmulateMediaOptions().setColorScheme(ColorScheme.DARK));
// Change media for page
page.emulateMedia(new Page.EmulateMediaOptions().setMedia(Media.PRINT));
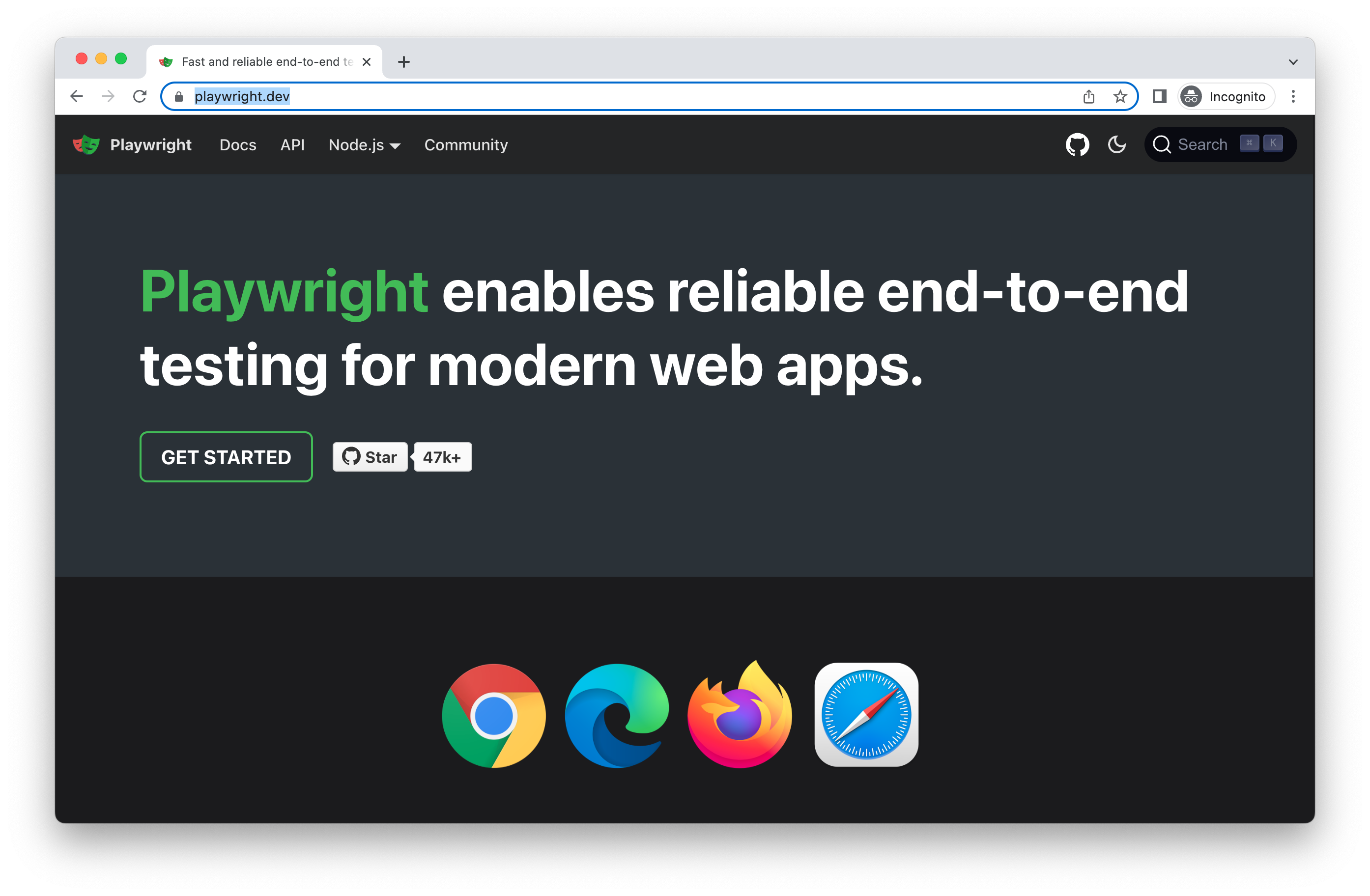
User Agent
The User Agent is included in the device and therefore you will rarely need to change it however if you do need to test a different user agent you can override it with the userAgent
property.
BrowserContext context = browser.newContext(new Browser.NewContextOptions()
.setUserAgent("My user agent"));
Offline
Emulate the network being offline.
BrowserContext context = browser.newContext(new Browser.NewContextOptions()
.setOffline(true));
JavaScript Enabled
Emulate a user scenario where JavaScript is disabled.
BrowserContext context = browser.newContext(new Browser.NewContextOptions()
.javaScriptEnabled(false));