Emulation
Introduction
With Playwright you can test your app on any browser as well as emulate a real device such as a mobile phone or tablet. Simply configure the devices you would like to emulate and Playwright will simulate the browser behavior such as "userAgent"
, "screenSize"
, "viewport"
and if it "hasTouch"
enabled. You can also emulate the "geolocation"
, "locale"
and "timezone"
for all tests or for a specific test as well as set the "permissions"
to show notifications or change the "colorScheme"
.
Devices
Playwright comes with a registry of device parameters using Playwright.Devices for selected desktop, tablet and mobile devices. It can be used to simulate browser behavior for a specific device such as user agent, screen size, viewport and if it has touch enabled. All tests will run with the specified device parameters.
using Microsoft.Playwright;
using System.Threading.Tasks;
using var playwright = await Playwright.CreateAsync();
await using var browser = await playwright.Chromium.LaunchAsync(new()
{
Headless = false
});
var iphone13 = playwright.Devices["iPhone 13"];
await using var context = await browser.NewContextAsync(iphone13);
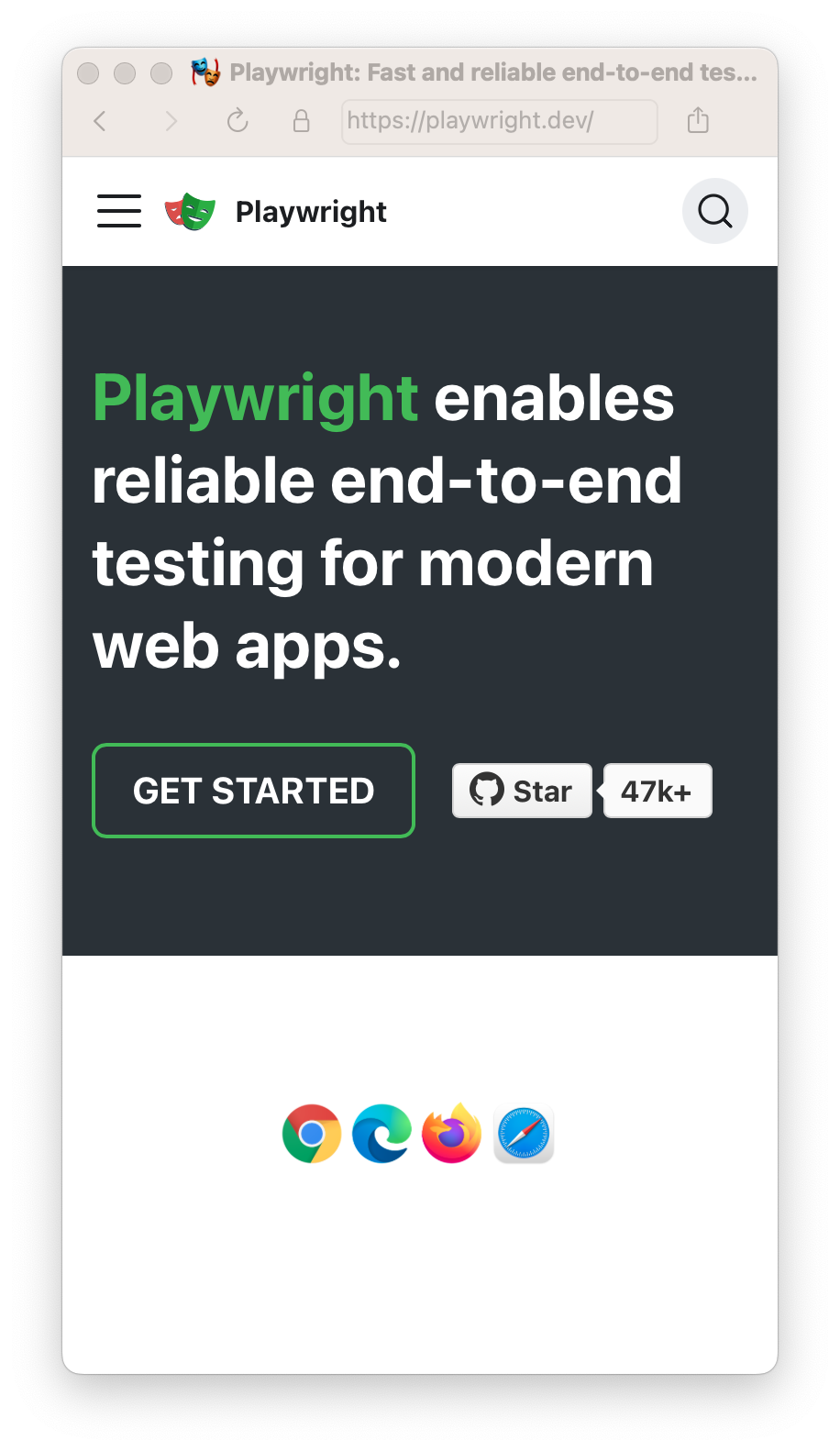
Viewport
The viewport is included in the device but you can override it for some tests with Page.SetViewportSizeAsync().
Test file:
The same works inside a test file.
// Create context with given viewport
await using var context = await browser.NewContextAsync(new()
{
ViewportSize = new ViewportSize() { Width = 1280, Height = 1024 }
});
// Resize viewport for individual page
await page.SetViewportSizeAsync(1600, 1200);
// Emulate high-DPI
await using var context = await browser.NewContextAsync(new()
{
ViewportSize = new ViewportSize() { Width = 2560, Height = 1440 },
DeviceScaleFactor = 2
});
isMobile
Whether the meta viewport tag is taken into account and touch events are enabled.
await using var context = await browser.NewContextAsync(new()
{
IsMobile = false
});
Locale & Timezone
Emulate the user Locale and Timezone which can be set globally for all tests in the config and then overridden for particular tests.
await using var context = await browser.NewContextAsync(new()
{
Locale = "de-DE",
TimezoneId = "Europe/Berlin"
});
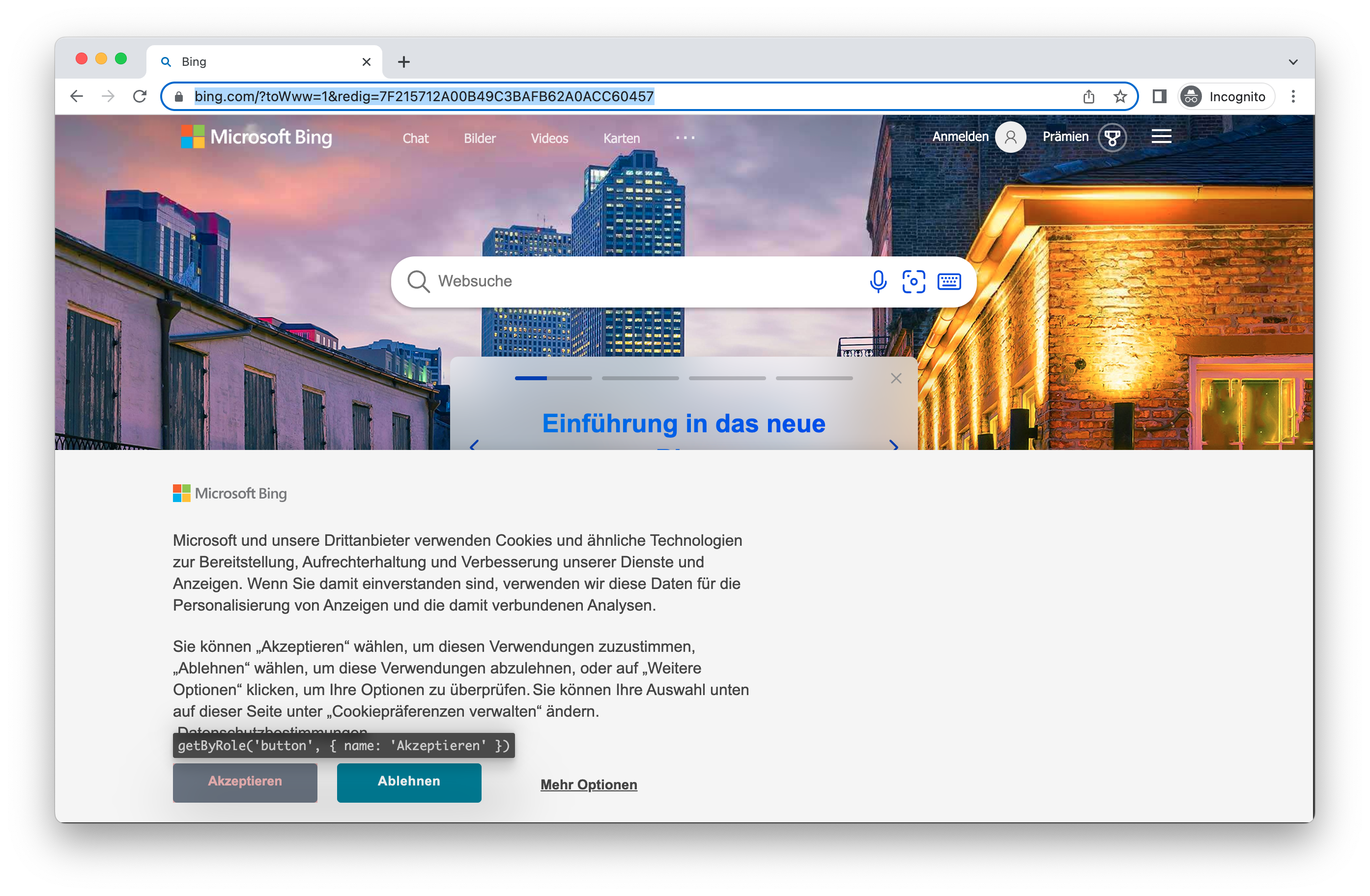
Permissions
Allow app to show system notifications.
Allow notifications for a specific domain.
await context.GrantPermissionsAsync(new[] { "notifications" }, origin: "https://skype.com");
Revoke all permissions with BrowserContext.ClearPermissionsAsync().
await context.ClearPermissionsAsync();
Geolocation
Grant "geolocation"
permissions and set geolocation to a specific area.
await using var context = await browser.NewContextAsync(new()
{
Permissions = new[] { "geolocation" },
Geolocation = new Geolocation() { Longitude = 41.890221, Latitude = 12.492348 }
});
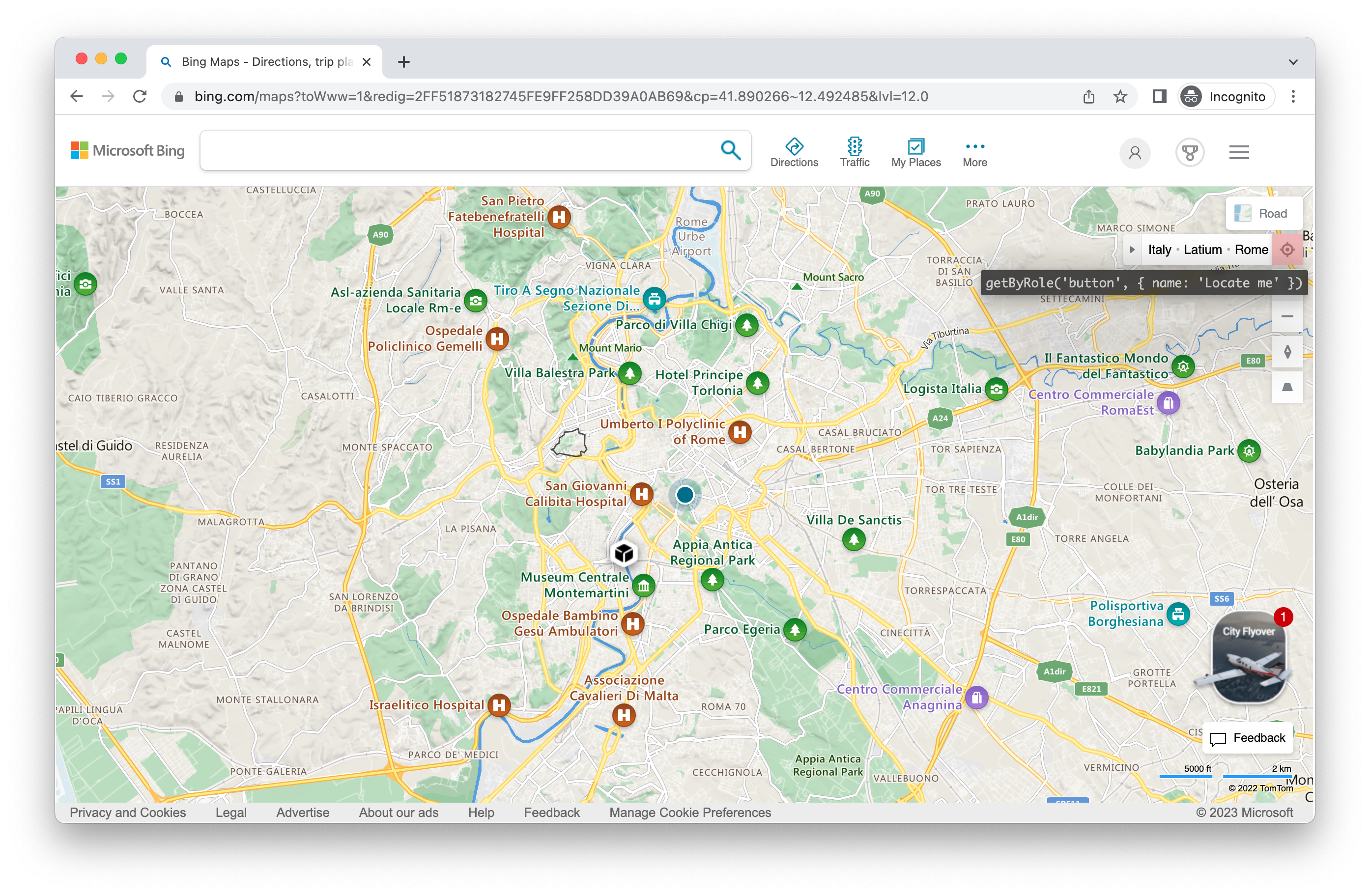
Change the location later:
await context.SetGeolocationAsync(new Geolocation() { Longitude = 48.858455, Latitude = 2.294474 });
Note you can only change geolocation for all pages in the context.
Color Scheme and Media
Emulate the users "colorScheme"
. Supported values are 'light', 'dark', 'no-preference'. You can also emulate the media type with Page.EmulateMediaAsync().
// Create context with dark mode
await using var context = await browser.NewContextAsync(new()
{
ColorScheme = ColorScheme.Dark
});
// Create page with dark mode
var page = await browser.NewPageAsync(new()
{
ColorScheme = ColorScheme.Dark
});
// Change color scheme for the page
await page.EmulateMediaAsync(new()
{
ColorScheme = ColorScheme.Dark
});
// Change media for page
await page.EmulateMediaAsync(new()
{
Media = Media.Print
});
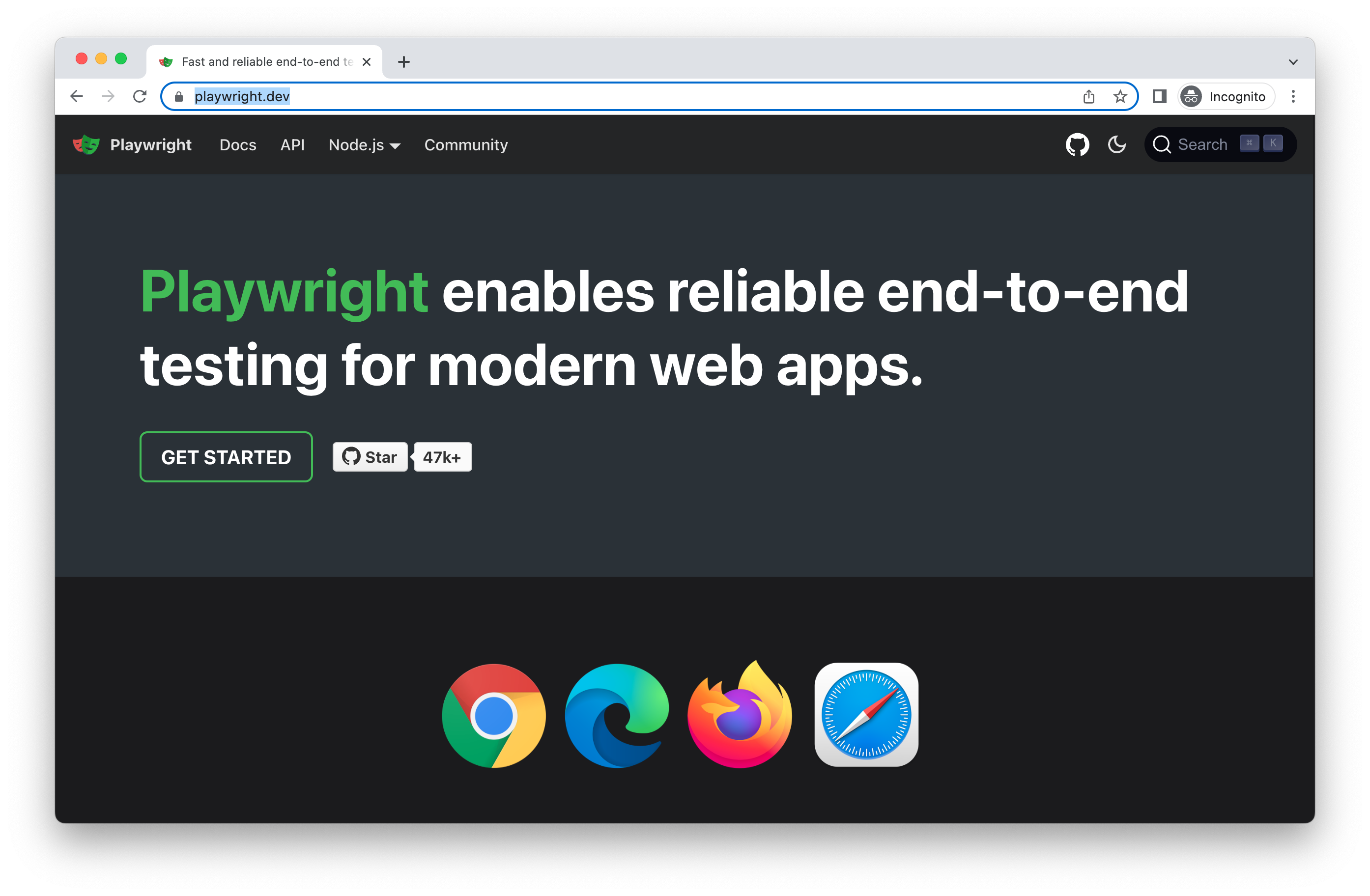
User Agent
The User Agent is included in the device and therefore you will rarely need to change it however if you do need to test a different user agent you can override it with the userAgent
property.
var context = await browser.NewContextAsync(new() { UserAgent = "My User Agent" });
Offline
Emulate the network being offline.
var context = await browser.NewContextAsync(new() { Offline = true });
JavaScript Enabled
Emulate a user scenario where JavaScript is disabled.
var context = await browser.NewContextAsync(new() { JavaScriptEnabled = false });